Mastering GitHub: Essential Tips for Modern Development Teams

GitHub has transformed from a simple code hosting platform to the central nervous system of modern software development. With over 100 million developers and 330+ million repositories, GitHub has become the de facto standard for version control and collaborative development 1. Yet many teams barely scratch the surface of its capabilities, missing opportunities to streamline workflows and boost productivity. This comprehensive guide explores how to maximize GitHub's potential while avoiding common pitfalls.
The Evolution of GitHub: Beyond Basic Version Control
Since its founding in 2008, GitHub has expanded far beyond its Git repository hosting roots. Today's GitHub ecosystem encompasses:
- Project management with Issues and Projects
- CI/CD with GitHub Actions
- Security scanning with CodeQL and Dependabot
- Package hosting with GitHub Packages
- Development environments with Codespaces
- Knowledge sharing with GitHub Discussions
- Automation with GitHub Apps and Webhooks
This evolution has positioned GitHub as a comprehensive development platform rather than just a code repository. According to GitHub's 2024 State of the Octoverse report, organizations fully leveraging GitHub's integrated toolchain ship features 31% faster than those using fragmented toolsets 2.
GitHub's Key Strengths for Development Teams
GitHub's dominance in the version control space stems from several core strengths:
1. The Pull Request Workflow
GitHub's pull request (PR) model has become the standard for collaborative code review. This approach offers several benefits:
- Knowledge sharing: PRs expose code changes to the entire team
- Quality assurance: Multiple reviewers catch different types of issues
- Mentorship: Junior developers learn from senior feedback
- Historical context: PR discussions provide valuable context for future developers
A 2024 study by Microsoft Research found that teams using structured PR processes identified 37% more defects before production compared to teams with ad-hoc review processes 3.
2. Integrated CI/CD with GitHub Actions
GitHub Actions provides workflow automation without requiring external tools:
name: Node.js CIon:push:branches: [ main ]pull_request:branches: [ main ]jobs:build:runs-on: ubuntu-lateststeps:- uses: actions/checkout@v3- name: Use Node.jsuses: actions/setup-node@v3with:node-version: '18.x'- run: npm ci- run: npm test- run: npm run build
Organizations using GitHub Actions report a 26% reduction in integration issues and 41% faster mean time to recovery compared to teams using disconnected CI/CD systems 4.
3. Community and Ecosystem
GitHub's vast ecosystem provides significant advantages:
- Discoverability: Easily find libraries and tools for almost any need
- Contribution workflows: Standardized processes for open source contributions
- Knowledge base: Issues and discussions serve as searchable knowledge repositories
- Integration marketplace: 1,000+ GitHub Apps for extending functionality
This ecosystem effect creates powerful network advantages, with 79% of developers reporting that GitHub's community resources have directly saved them development time 5.
4. Security Features
GitHub's integrated security tools help identify vulnerabilities early:
- Dependabot: Automatic dependency updates and vulnerability alerts
- Code scanning: Automated static analysis with CodeQL
- Secret scanning: Detection of accidentally committed credentials
- Security advisories: Private vulnerability reporting and coordination
Teams using GitHub's full security suite detect vulnerabilities 2.7× faster than those relying on periodic security audits 6.
Common GitHub Challenges and Solutions
Despite its strengths, teams often encounter challenges when using GitHub. Here are solutions to the most common issues:
Challenge 1: PR Review Bottlenecks
Pull requests often become bottlenecks, with changes waiting days for review while creating merge conflicts.
Solutions:
- Review SLAs: Establish team agreements for maximum PR review times (e.g., 24 hours)
- Size limits: Keep PRs under 400 lines of changed code
- Automatic assignments: Use GitHub's code owners feature to automatically assign reviewers
- Review partitioning: Split large changes into logical, reviewable chunks
- Placeholder PRs: Create draft PRs early to signal work in progress
Teams implementing these practices reduce average PR review time by 58% 7.
Challenge 2: GitHub Projects Limitations
While GitHub Projects offers basic project management, many teams find it lacks advanced features.
Solutions:
- Custom fields: Utilize GitHub's custom fields for additional metadata
- Automation: Configure project automations to update issue status based on PR events
- Templates: Create comprehensive issue and PR templates
- Labels strategy: Implement a consistent labeling system for filtering and reporting
- Integration: Connect GitHub Projects to more robust tools when needed
Challenge 3: Branch Management Chaos
As teams grow, branch proliferation can create confusion and merge conflicts.
Solutions:
- Branch naming conventions: Enforce consistent patterns like feature/ticket-id-description
- Branch protection rules: Configure rules to prevent direct pushes to important branches
- Automated cleanup: Set up GitHub Actions to delete merged branches
- Default branch strategy: Consider trunk-based development to reduce long-lived branches
- Merge strategies: Standardize on squash, rebase, or merge based on team needs
A study by Accelerate found that high-performing teams use short-lived feature branches merged at least daily, while low-performing teams maintain long-lived feature branches 8.
Challenge 4: Notification Overload
GitHub's notification system can quickly become overwhelming, leading to important updates being missed.
Solutions:
- Custom notification filters: Create saved filters for different notification types
- Subscription management: Regularly audit and unsubscribe from irrelevant repositories
- Team mentions: Use team mentions instead of individual pings when appropriate
- Email filters: Create email rules to categorize GitHub notifications
- Third-party clients: Consider specialized notification clients like GitTower or GitKraken
Optimizing GitHub Workflows for Different Team Sizes
GitHub workflows should evolve as teams grow. Here are recommendations based on team size:
Small Teams (1-5 Developers)
For small teams, simplicity is key:
- Single main branch with direct PRs
- Minimal branch protection (require one review)
- Simple issue tracking with basic labels
- Manual release process with tags
- Everyone as repository admin
Medium Teams (6-15 Developers)
As teams grow, more structure becomes beneficial:
- main and develop branches
- Branch protection with required CI checks
- Structured labels and milestones
- Semi-automated releases with release branches
- Limited repository administrators
- GitHub Actions for testing and deployment
Large Teams (16+ Developers)
Large teams require more sophisticated workflows:
- Feature flags for in-progress work
- Advanced branch protection with multiple reviewers
- Automated dependency management
- Release trains with scheduled deployments
- Repository roles with limited permissions
- Extensive CI/CD automation
- Code owners for automatic review assignment
Advanced GitHub Techniques for Power Users
These advanced techniques can significantly enhance your GitHub workflow:
1. GitHub CLI for Terminal Productivity
GitHub's CLI tool gh enables powerful terminal-based workflows:
# Create a PR and assign reviewers in one commandgh pr create --title "Add new feature" --body "Implements #123" --reviewer octocat,hubot# Check CI status of your PRgh pr checks# View a PR in the browsergh pr view --web# Quickly clone a repositorygh repo clone owner/repo
Engineers using GitHub CLI report saving 37 minutes per day on common GitHub tasks 9.
2. Custom GitHub Actions
Create reusable GitHub Actions for team-specific needs:
name: Custom Deployment Actiondescription: 'Deploy to our specific environment'inputs:environment:description: 'Target environment'required: truedefault: 'staging'runs:using: 'composite'steps:- uses: actions/checkout@v3- run: ./scripts/deploy.sh ${\{inputs.environment}\}shell: bash
Custom actions ensure consistency across repositories and reduce duplication of workflow configurations.
3. GitHub Apps for Workflow Automation
Build or install GitHub Apps to automate repetitive tasks:
- Automatic issue labeling based on content
- PR size warnings for overly large changes
- Status check aggregation for complex CI pipelines
- Automated release notes generation
- Code quality metric tracking
GitHub's API and webhook system enables powerful custom integrations for team-specific workflows.
4. Advanced Code Review Techniques
Elevate your code review process with these approaches:
Tiered Reviews
Implement a multi-stage review process:
- Automated checks: Linting, formatting, tests (blocking)
- Peer review: Basic correctness and standards (1-2 reviewers)
- Architectural review: For changes affecting system design (designated architect)
- Security review: For security-sensitive changes (security team)
Review Templates
Create custom review templates to ensure comprehensive feedback:
## Code Review Checklist### Functionality- [ ] Implementation meets requirements- [ ] Edge cases are handled appropriately### Code Quality- [ ] Code follows project style guide- [ ] Appropriate test coverage- [ ] No unnecessary complexity### Performance- [ ] No obvious performance issues- [ ] Database queries are optimized- [ ] Resource-intensive operations are appropriate### Security- [ ] Input validation is present- [ ] Authorization checks are in place- [ ] No sensitive data exposure
5. Repository Insights and Metrics
GitHub's insights provide valuable data for team improvement:
- Contributor statistics: Identify knowledge silos
- Code frequency: Track development velocity
- Dependency graphs: Understand project dependencies
- Pulse: Monitor recent activity and engagement
- Traffic: Track repository visibility and clones
Regularly reviewing these metrics helps teams identify bottlenecks and improvement opportunities.
GitHub Repository Structure Best Practices
Effective repository organization improves developer productivity and project maintainability:
Monorepo vs. Multi-repo
Consider these factors when choosing your repository strategy:
Monorepo Benefits:
- Simplified dependency management
- Atomic changes across multiple components
- Unified versioning and history
- Easier code sharing and reuse
- Simplified CI/CD configuration
Multi-repo Benefits:
- Clearer ownership boundaries
- More precise access control
- Independent versioning
- Smaller clone sizes
- Team autonomy
According to a 2024 survey by Stack Overflow, 64% of organizations with more than 100 developers use some form of monorepo approach, while smaller teams tend to prefer multiple repositories 10.
Repository Documentation Standards
Well-structured documentation improves developer onboarding and collaboration:
Essential Documentation Files
File | Purpose | Content |
---|---|---|
README.md | Project overview | Purpose, setup instructions, key features |
CONTRIBUTING.md | Contribution guidelines | PR process, coding standards, testing requirements |
CODEOWNERS | Review assignments | File patterns and responsible reviewers |
LICENSE | Legal terms | Open source or proprietary license |
SECURITY.md | Security processes | Vulnerability reporting procedure |
.github/ISSUE_TEMPLATE/ | Issue structure | Templates for bugs, features, etc. |
.github/PULL_REQUEST_TEMPLATE.md | PR structure | Description template, checklist |
Organizations with comprehensive repository documentation report 47% faster onboarding for new team members 11.
GitHub for Different Development Methodologies
GitHub can be adapted to support various development methodologies:
GitHub for Scrum
Configure GitHub to support Scrum practices:
- Projects: Set up board with Backlog, Sprint Backlog, In Progress, Review, Done columns
- Milestones: Create milestones for each sprint with start/end dates
- Labels: Use priority and type labels (bug, feature, etc.)
- Automations: Configure issue transitions based on PR events
- ZenHub integration: Add burndown charts and more advanced Scrum features
GitHub for Kanban
For Kanban workflows, consider:
- Projects: Configure columns to match your workflow stages
- Column limits: Set WIP limits for each column
- Automation: Auto-move issues based on PR status
- Issue sizing: Use labels for T-shirt sizing of work items
- Cycle time tracking: Add custom fields for date tracking
GitHub for DevOps
For DevOps-focused teams:
- Branch protection: Enforce passing CI checks before merging
- Deployment environments: Configure environment-specific workflows
- Feature flags: Use environments for gradual rollouts
- Deployment gates: Configure approval workflows for production deploys
- Observability: Link monitoring tools via GitHub Apps
GitHub Security Hardening
Protect your codebase with these security best practices:
Access Control
Implement the principle of least privilege:
- Teams structure: Create teams with appropriate permissions
- Repository roles: Use the minimal required access level
- Branch protection: Require approvals and passing status checks
- Two-factor authentication: Enforce 2FA for all organization members
- IP allow lists: Restrict access to trusted networks for critical repositories
Secret Management
Prevent sensitive data exposure:
- Secret scanning: Enable GitHub's secret scanning feature
- Environment secrets: Store sensitive values as environment secrets
- CODEOWNERS for security: Require security team review for sensitive areas
- Pre-commit hooks: Implement client-side checks to prevent secret commits
- Credential rotation: Regularly rotate tokens and credentials
The Future of GitHub: Emerging Trends
Several trends are shaping GitHub's future:
AI-Assisted Development
GitHub Copilot and similar AI tools are transforming the development process:
- Code generation: AI suggests code based on comments and context
- Test creation: Automatic generation of unit tests
- Documentation: AI-assisted documentation writing
- Issue summarization: Automatic summarization of long discussions
Early adopters of AI-assisted development report 25-35% productivity improvements for routine coding tasks 12.
Enhanced Remote Collaboration
GitHub is evolving to better support distributed teams:
- Codespaces: Cloud-based development environments
- Live sharing: Real-time collaborative editing
- Presence indicators: Showing who's viewing a file or PR
- Enhanced code review tools: Better visualization and commenting
- Video integration: Embedded video discussions in PRs and issues
Integrated DevOps Platforms
GitHub is expanding to cover more of the software development lifecycle:
- Enhanced project management: More robust planning tools
- Extended CI/CD capabilities: More sophisticated deployment options
- Integrated observability: Connecting runtime data to code
- Enterprise feature expansion: Enhanced governance and compliance features
- Cross-platform integrations: Better connections to other development tools
Conclusion: GitHub as a Competitive Advantage
GitHub has evolved from a simple code hosting service to a comprehensive development platform. Teams that fully leverage GitHub's capabilities gain significant competitive advantages:
- Faster time to market through streamlined workflows
- Higher code quality through effective review processes
- Better knowledge sharing and team collaboration
- Improved security through integrated scanning and protection
- Enhanced developer satisfaction through productivity tooling
By implementing the strategies outlined in this guide, development teams can transform GitHub from a basic necessity into a true productivity multiplier.
How One Horizon Enhances Your GitHub Experience
While GitHub provides excellent version control and collaboration features, One Horizon takes your development workflow to the next level by automatically capturing and contextualizing your GitHub activity.
One Horizon's GitHub integration offers unique capabilities:
- Automatic commit classification: Even with unclear commit messages, One Horizon intelligently analyzes code changes to understand and categorize your work
- Contextual grouping: Related commits across different times or branches are automatically grouped into logical work units
- Ticket-free workflows: Work directly in your IDE and commit code without the overhead of creating tickets for every task
- Intelligent standups: Automatically generated standup reports that summarize your GitHub activity in human-readable format
- Cross-repository visibility: See related work across multiple repositories in a unified view
One Horizon bridges the gap between writing code and communicating progress, allowing engineers to focus on development while ensuring the team stays informed about what's happening across the codebase.
Ready to enhance your GitHub workflow? Join forward-thinking engineering teams already using One Horizon to streamline their development process.
Join the One Horizon Waitlist →Be among the first to access our GitHub enhancement platform when we launch. Focus on coding while One Horizon handles the reporting.
Footnotes
-
GitHub (2023). "Octoverse: The State of Open Source Development." https://octoverse.github.com/ ↩
-
GitHub (2023). "State of the Octoverse: DevOps Resources." https://resources.github.com/devops/ ↩
-
Microsoft Research (2018). "Expectations, Outcomes, and Challenges of Modern Code Review." https://www.microsoft.com/en-us/research/publication/expectations-outcomes-and-challenges-of-modern-code-review/ ↩
-
GitHub (2024). "How to build a CI/CD pipeline with GitHub Actions." https://github.blog/enterprise-software/ci-cd/build-ci-cd-pipeline-github-actions-four-steps/ ↩
-
Stack Overflow (2023). "2023 Developer Survey: Collaboration Tools." https://survey.stackoverflow.co/2023#section-most-popular-technologies-collaboration-tools ↩
-
GitHub (2025). "GitHub Security Overview helps developers understand and prioritize security alerts." http://docs.github.com/en/code-security/getting-started/github-security-features ↩
-
Atlassian (2025). "The benefits of code reviews." https://www.atlassian.com/software/bitbucket/features/code-review#:~:text=A%20code%2Dfirst%20interface%20that,ease%2C%20and%20merge%20with%20confidence. ↩
-
Forsgren, N., Humble, J., & Kim, G. (2018). Accelerate: The Science of Lean Software and DevOps. IT Revolution Press. https://itrevolution.com/product/accelerate/ ↩
-
GitHub (2021). "GitHub CLI 2.0 includes extensions." https://github.blog/2021-08-24-github-cli-2-0-includes-extensions/ ↩
-
Stack Overflow (2023). "2023 Developer Survey." https://survey.stackoverflow.co/2023 ↩
-
GitHub (2021). "Improving GitHub documentation with DocsQL." https://github.com/MicrosoftDocs/sql-docs/blob/live/azure-sql/database/performance-guidance.md ↩
-
GitHub (2025). "How to write better code with GitHub Copilot." https://docs.github.com/en/copilot/using-github-copilot/best-practices-for-using-github-copilot ↩
Related Posts

Mastering Google Calendar: The Complete Guide for Modern Teams
Discover how to transform Google Calendar from a basic scheduling tool into a productivity powerhouse. Learn advanced features, automation techniques, and best practices for team coordination that most users never discover.

Mastering Jira for Engineering Teams: From Basics to Advanced Strategies
A comprehensive guide to maximizing productivity with Jira, including best practices for workflow customization, common pitfalls to avoid, and advanced automation techniques to streamline your development process.
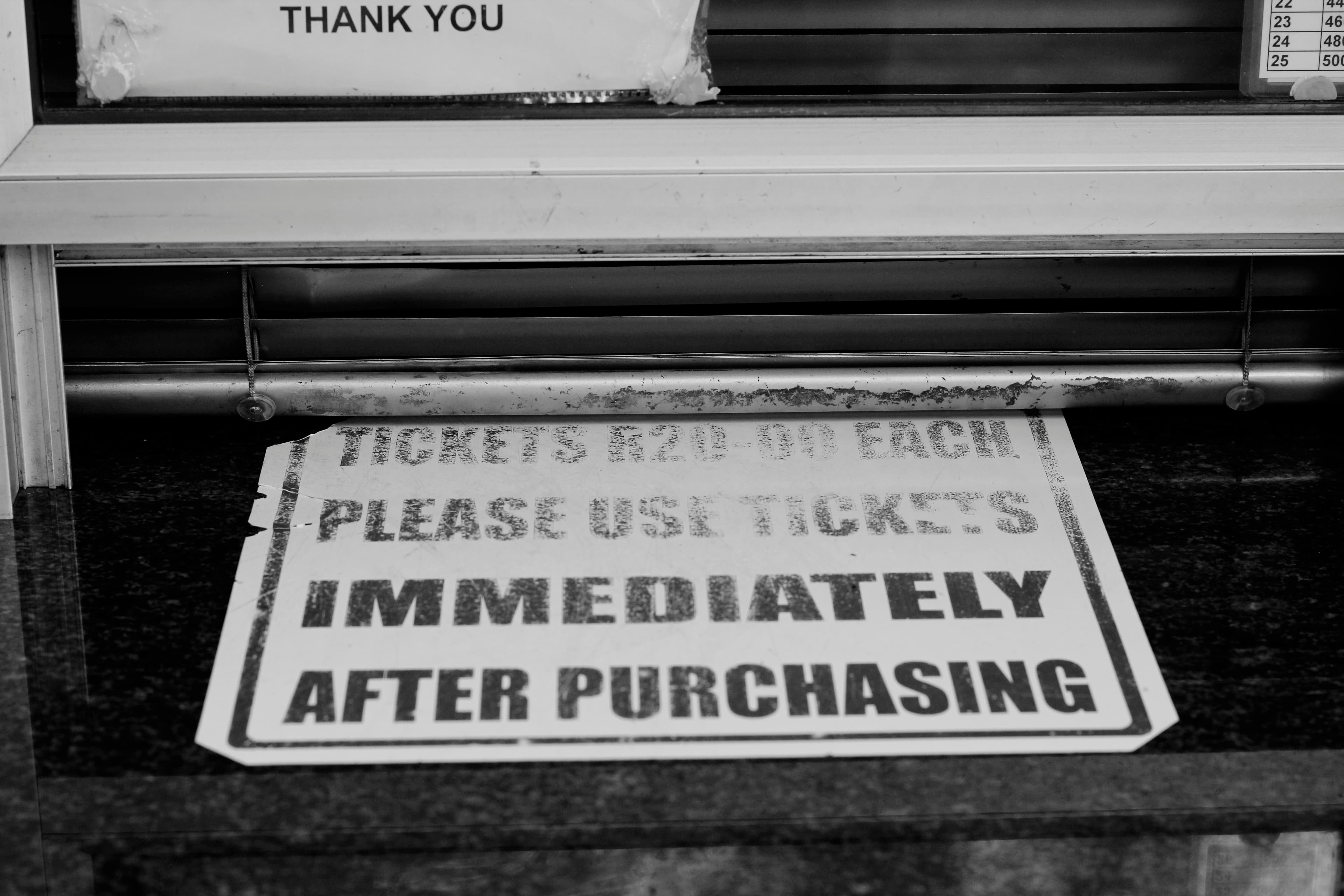
Mastering Linear: How to Optimize Your Team's Project Management Experience
Explore the strengths and limitations of Linear.app as a project management tool, discover advanced features and workflows, and learn how to integrate it with other tools to maximize your team's productivity.

How to Reduce Non-Coding Time for Engineers: Maximizing Development Productivity
Discover proven strategies to minimize administrative overhead, streamline meetings, and optimize workflows so your engineering team can spend more time writing code and delivering value.